- Serialization is the process of saving the objects loaded in the memory to corresponding streams
- e.g Saving the objects to file system.
- Deserialization is the process of loading the saved data to corresponding objects.
- We have already discussed about the basics of serialization and deserialization process.
What Serializable interface?
- Serializable is a marker interface.
- Serializable interface does not contain any method.
- Serializable interface is used to inform the JVM that class wants serialization feature.
public interface Serializable { }
Serialize the object or pojo in java using Serializable interface
1.) Person class or POJO class:
- We would like to serialize the Person class.
- The person class should implement the Serializable interface.
- We will use following classes for Serialization & Deserialization:
- ObjectOutputStream will be used to serialize person object.
- ObjectInputStream will be used to deserialize person object.
import java.io.Serializable; public class Person implements Serializable { private static final long serialVersionUID = 1L; public String firstName; public String lastName; public int age; public String contact; public Person(String firstName, String lastName, int age, String contact) { this.firstName = firstName; this.lastName = lastName; this.age = age; this.contact = contact; } public String toString() { return "[" + firstName + " " + lastName + " " + age + " " +contact +"]"; } }
2.) SerializeDeserialize class:
We have written couple of methods to serialize and deserialize person objects.
- serialize method : serialize method will save person object to “savePerson.txt” file.
- serialize method will prints the serialized person object.
- deserialize method: deserialize method will deserialize person object saved in savePerson.txt file
- deserialize method, prints the deserialized person object.
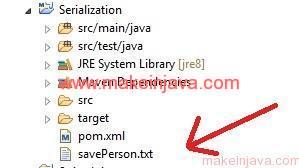
import java.io.File; import java.io.FileInputStream; import java.io.FileOutputStream; import java.io.IOException; import java.io.ObjectInputStream; import java.io.ObjectOutputStream; public class SerializeDeserialize { public static void serialize() throws IOException { Person person = new Person("Mike", "harvey", 34, "001894536"); FileOutputStream output = new FileOutputStream(new File("savePerson.txt")); ObjectOutputStream outputStream = new ObjectOutputStream(output); outputStream.writeObject(person); output.close(); outputStream.close(); System.out.println("Serialized the person object : "+person); } public static void deSerialize() throws IOException, ClassNotFoundException { FileInputStream inputStream = new FileInputStream(new File("savePerson.txt")); ObjectInputStream objectInputStream = new ObjectInputStream(inputStream); Person person = (Person) objectInputStream.readObject(); System.out.println("Deserialize the person object :"+ person); objectInputStream.close(); inputStream.close(); } public static void main(String[] args) throws IOException, ClassNotFoundException { serialize(); deSerialize(); } }
Output – Serialize & deserialize person object or POJO in java
Serialized the person object : [Mike harvey 34 001894536] Deserialize the person object :[Mike harvey 34 001894536]
Download code – Serialization & deserialization object in java