- Read & Write file in java using InputStreamReader & OutputStreamWriter class.
- We will write contents to a file using OutputStreamWriter class in java.
- We will read contents from file using InputStreamReader class in java.
- InputStreamReader and OutputStreamWriter is character based reader and writer.
1. InputStreamReader class:
- InputStreamReader converts bytes to characters using specified charset.
- Read methods InputStreamReader class are as follows:
No. | Method prototype | Description |
---|---|---|
1 | int read() | Reads a single character. |
2 | int read(char[] cbuf, int offset, int length) | Reads characters into a portion of an array. |
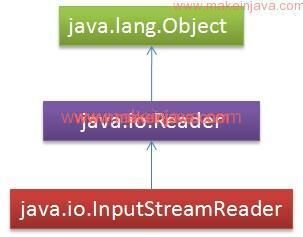
2. OutputStreamWriter class:
- OutputStreamWriter converts characters to bytes using specified charset.
- Write methods of OutputStreamWriter class are as follows:
No. | Method prototype | Description |
---|---|---|
1 | void write(char[] cbuf, int off, int len) | Writes a portion of an array of characters. |
2 | void write(int c) | Writes a single character. |
3 | void write(String str, int off, int len) | Writes a portion of a string. |
OutputStreamWriter will act as character to byte encoder and InputStreamReader will act as byte to character decoder.
3. Read & write file in java (InputStreamReader/OutputStreamWriter)
package org.learn.io; import java.io.File; import java.io.FileInputStream; import java.io.FileOutputStream; import java.io.IOException; import java.io.InputStreamReader; import java.io.OutputStreamWriter; public class SteamReaderWriter { public static void main(String[] args) throws IOException { //Write contents to file using input stream writer writeUsingStreamWriter(); //Read contents from file using input stream reader readUsingStreamReader(); } private static void writeUsingStreamWriter() throws IOException { String newLine = System.getProperty("line.separator"); try (FileOutputStream fileStream = new FileOutputStream( new File("sampleFile.txt")); OutputStreamWriter writer = new OutputStreamWriter(fileStream)) { System.out.println("1. Start Writing file using OutputStreamWriter:"); writer.write("Soccer"); System.out.println("Written \"Soccer\" to a file"); writer.write(newLine); writer.write("Tennis"); System.out.println("Written \"Tennis\" to a file"); writer.write(newLine); writer.write("Badminton"); System.out.println("Written \"Badminton\" to a file"); writer.write(newLine); writer.write("Hockey"); System.out.println("Written \"Hockey\" to a file"); writer.write(newLine); System.out.println("2. End Writing file using OutputStreamWriter"); System.out.println(); } } private static void readUsingStreamReader() throws IOException { try (FileInputStream fileStream = new FileInputStream( new File("sampleFile.txt")); InputStreamReader reader = new InputStreamReader(fileStream)) { System.out.println("3. Start Reading the file using InputStreamReader:"); int character; while ((character = reader.read()) != -1) { System.out.print((char) character); } System.out.println("4. End Reading the file using InputStreamReader"); } } }
The content of sampleFile.txt is as follows:
4. O/P:Read & write file in java – InputStreamReader & OutputStreamWriter
1. Start Writing file using OutputStreamWriter: Written "Soccer" to a file Written "Tennis" to a file Written "Badminton" to a file Written "Hockey" to a file 2. End Writing file using OutputStreamWriter 3. Start Reading the file using InputStreamReader: Soccer Tennis Badminton Hockey 4. End Reading the file using InputStreamReader