- Linked list in java is a doubly-linked list.
- Insertion order is maintained in a linked list.
- LinkedList extends AbstractSequentialList class & implements List, Deque, Cloneable, Serializable interfaces.
- LinkedList class is not thread safe.
- In multi threaded environment, it should be synchronized externally.
- The iterators returned by LinkedList class’s iterator and listIterator methods are fail-fast.
- LinkedList class can contain duplicate elements.
We would like to iterate or loop through the linkedlist collection of String objects in java.
1. LinkedList collection class hierarchy:
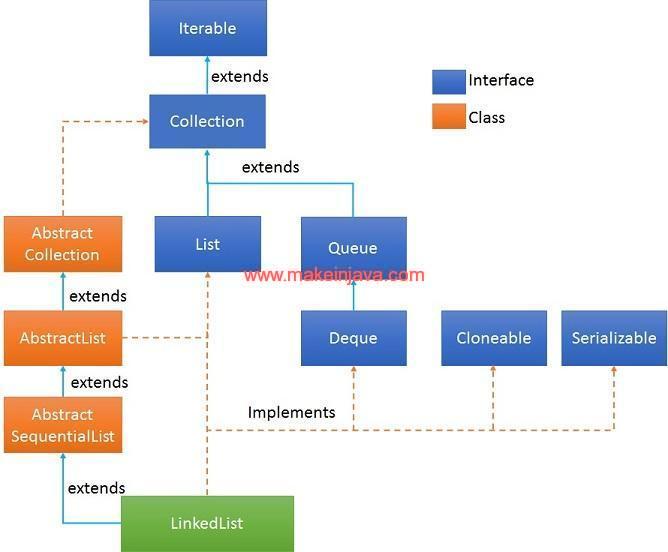
2. Iterate or loop LinkedList collection of String objects in java (example)
package org.learn.collection.list.linkedlist; import java.util.LinkedList; import java.util.ListIterator; public class DemoIterationArraylist { public static void main(String[] args) { LinkedList<String> linkedList = new LinkedList<>(); linkedList.add("archery"); linkedList.add("badminton"); linkedList.add("canoe"); linkedList.add("boxing"); linkedList.add("diving"); System.out.println("\nDemo: Iterate or loop LinkedList collection: "); demoIterateMethod(linkedList); } private static void demoIterateMethod(LinkedList<String> linkedList) { System.out.println("1. Iterating using forEachRemaining:"); // Output of for loop: // golf diving archery linkedList.forEach(element -> System.out.printf("%s ", element)); System.out.println("\n2. Iterating linkedList using foreach loop:"); // Output of for loop: // golf diving archery for (String element : linkedList) { System.out.printf("%s ", element); } System.out.println("\n3. Iterating linkedList using iterator:"); ListIterator<String> listIterator = linkedList.listIterator(); // Output of while loop: // golf diving archery while (listIterator.hasNext()) { System.out.printf("%s ", listIterator.next()); } System.out.println(""); } }
3. Output: Iterate LinkedList collection of String objects (java /example)
Demo: Iterate or loop LinkedList collection: 1. Iterating using forEachRemaining: archery badminton canoe boxing diving 2. Iterating linkedList using foreach loop: archery badminton canoe boxing diving 3. Iterating linkedList using list iterator: archery badminton canoe boxing diving