- Given the UTF contents, read & write utf contents using InputStreamReader & OutputStreamWriter.
- We have already discussed similar posts:
- Read & write UTF file – BufferReader & BufferWriter (using NIO)
- Read & write contents (without UTF) using InputStreamReader/OutputStreamWriter
In this post, we will demonstrate read and write operation with UTF-8 contents.
Read write utf file – InputStreamReader & OutputStreamWriter
package org.learn.utf.io; import java.io.File; import java.io.FileInputStream; import java.io.FileOutputStream; import java.io.IOException; import java.io.InputStreamReader; import java.io.OutputStreamWriter; public class SteamReaderWriter { public static void main(String[] args) throws IOException { writeUTFUsingStreamWriter(); readUTFUsingStreamReader(); } private static void writeUTFUsingStreamWriter() throws IOException { System.out.println( "1. Start writting contents to file - OutputStreamWriter" ); String newLine = System.getProperty( "line.separator" ); try (FileOutputStream fileStream = new FileOutputStream( new File( "sampleUTFFile.txt" )); OutputStreamWriter writer = new OutputStreamWriter(fileStream, "UTF-8" )) { writer.write( "Names of Jackie Chan:" ); writer.write(newLine); writer.write( "房仕龍 (Fong Si-lung)" ); writer.write(newLine); writer.write( "元樓 (Yuen Lou)" ); writer.write(newLine); writer.write( "大哥 (Big Brother)" ); writer.write(newLine); } System.out.println( "2. Successfully written contents to file - OutputStreamWriter" ); } private static void readUTFUsingStreamReader() throws IOException { try (FileInputStream fileStream = new FileInputStream( new File( "sampleUTFFile.txt" )); InputStreamReader reader = new InputStreamReader(fileStream, "UTF-8" )) { System.out.println( "\n3. Start Reading file using InputStreamReader:" ); int character; while ((character = reader.read()) != - 1 ) { System.out.print(( char ) character); } System.out.println( "4. End Reading file using InputStreamReader" ); } } } |
The content of sampleUTFFile.txt is as follows:
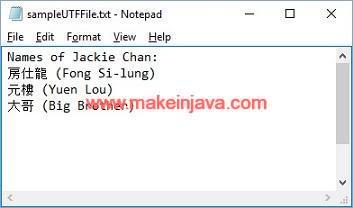
O/P: Read write file – InputStreamReader & OutputStreamWriter
1. Start writting contents to file - OutputStreamWriter 2. Successfully written contents to file - OutputStreamWriter 3. Start Reading file using InputStreamReader: Names of Jackie Chan: 房仕龍 (Fong Si-lung) 元樓 (Yuen Lou) 大哥 (Big Brother) 4. End Reading file using InputStreamReader |