- Given any web page, we have a functionality to drag and drop an item from one location to another location. Selenium API has given no direct method for handling drag and drop functionality. However, Selenium web driver has provided an “Actions” class to handle this kind of scenarios. Lets discuss how to use Actions class to handle this scenario in detail.
- Actions class also provides features like Mouseover, Enter Text in Capital letters, Right Click, DoubleClick etc.
Actions class provides two methods for handling drag & drop:
- dragAndDrop(Sourcelocator, Destinationlocator) :
- Parameters: “Sourcelocator” is the element we need to drag , “Destinationlocator” is the element on which we need to drop the first element(SourceLocator).
- dragAndDropBy(source, xOffset, yOffset) :
- This method performs like click-and-hold at the location of the source element, moves by a given offset value, then releases the mouse. In this method, we needs to find the pixels of target element. However, pixel values keep on changing with respect to screen resolution and browser dimensions. Hence, this method is neither reliable nor widely used.
- Parameters: “source element” to select the element we want to drag, “xOffset” horizontal move offset, “yOffset” vertical move offset.
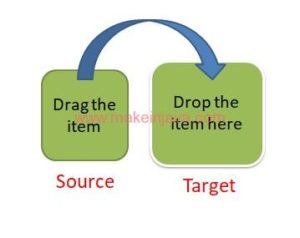
Code : Drag & Drop using dragAndDrop(Sourcelocator, Destinationlocator):
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.interactions.Actions;
import org.testng.annotations.Test;
public class DragAndDrop {
WebDriver driver;
@Test
public void DragAndDropTest()
{
System.setProperty("webdriver.chrome.driver","C://chromedriver.exe ");
driver= new ChromeDriver();
driver.get("Enter the URL of the Website");
//Element needs to drag.
WebElement sourceElement =driver.findElement(By.xpath("enter xpath here"));
//Element on which need to drop.
WebElement targetElement =driver.findElement(By.xpath("enter xpath here"));
// Create object of Actions class for drag and drop.
Actions actions = new Actions(driver);
//Drag and drop method.
actions.dragAndDrop(sourceElement, targetElement ).build().perform();
}
}
Code : Drag & Drop using dragAndDropBy(source, xOffset, yOffset):
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.interactions.Actions;
import org.testng.annotations.Test;
public class DragAndDrop {
WebDriver driver;
@Test
public void DragAndDropTest() {
System.setProperty("webdriver.chrome.driver", " C://chromedriver.exe ");
driver = new ChromeDriver();
driver.get("Enter the URL of the Website");
// Element needs to drag.
WebElement sourceElement = driver.findElement(By.xpath("enter xpath here"));
// Create object of Actions class for drag and drop.
Actions actions = new Actions(driver);
// Drag and Drop by Pixel
actions.dragAndDropBy(sourceElement, 150, 50).build().perform();
}
}