What is Java Bank ATM Simulator?
Java Bank ATM Simulator, an interactive program designed for simulating banking transactions. Java-based system offers a hands-on experience in managing finances. With its intuitive interface, we can effortlessly navigate through checking your balance, making withdrawals, and depositing funds. It’s like having a virtual bank at our fingertips, enabling you to practice responsible financial management in a risk-free environment.
Gain practical insights into handling money effectively by using this Java-powered program. Discover the ease of monitoring your balance, conducting transactions, and learning essential financial skills.
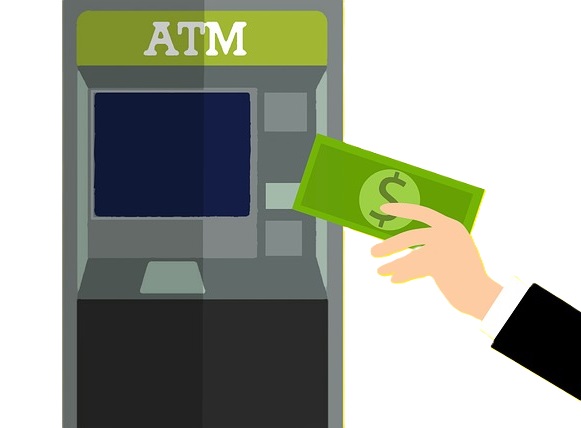
Approach to Bank ATM Simulator to manage finances?
- Create an ATM class which will manage banking operations.
- Operations supported by ATM Bank Simulator
- Check Balance
- Withdraw Money from ATM
- Deposit Money to ATM
- Set an initial balance (e.g., $1000) in Bank Account on initialization.
- We will use Java Scanner class to take inputs from user.
- Implement operations or functions supported by ATM Bank Simulator
- Check balance (checkBalance()).
- Withdraw funds (withdrawFunds()).
- Deposit funds (depositFunds()).
- Develop a Loop for for operations until user exits:
- Check Balance, Withdraw, Deposit, Exit.
Program: Develop Bank ATM Simulator in Java
import java.util.Scanner; public class BankATMSimulator { private static double balance = 1000 ; // initial balance public static void main(String[] args) { Scanner scanner = new Scanner(System.in); while ( true ) { System.out.println( "Bank ATM Simulator Choices:" ); System.out.println( "1. Check Balance" ); System.out.println( "2. Withdraw Funds" ); System.out.println( "3. Deposit Funds" ); System.out.println( "4. Exit" ); System.out.print( "Enter your choice: " ); int choice = scanner.nextInt(); switch (choice) { case 1 : checkBalance(); break ; case 2 : withdrawFunds(scanner); break ; case 3 : depositFunds(scanner); break ; case 4 : System.out.println( "Thank you for using the Bank ATM. Goodbye!" ); System.exit( 0 ); default : System.out.println( "Invalid choice. Please enter a valid option." ); break ; } } } private static void checkBalance() { System.out.println( "Your current balance is: $" + balance); } private static void withdrawFunds(Scanner scanner) { System.out.print( "Enter the amount to withdraw: $" ); double amount = scanner.nextDouble(); if (amount > balance) { System.out.println( "Insufficient funds. Withdrawal cannot be processed." ); } else { balance -= amount; System.out.println( "Withdrawal of $" + amount + " successful. Remaining balance: $" + balance); } } private static void depositFunds(Scanner scanner) { System.out.print( "Enter the amount to deposit: $" ); double amount = scanner.nextDouble(); if (amount <= 0 ) { System.out.println( "Invalid amount. Deposit cannot be processed." ); } else { balance += amount; System.out.println( "Deposit of $" + amount + " successful. New balance: $" + balance); } } } |
Output: Bank ATM Simulator in Java
Bank ATM Simulator Choices: 1 . Check Balance 2 . Withdraw Funds 3 . Deposit Funds 4 . Exit Enter your choice: 1 Your current balance is: $ 1000.0 Bank ATM Simulator Choices: 1 . Check Balance 2 . Withdraw Funds 3 . Deposit Funds 4 . Exit Enter your choice: 2 Enter the amount to withdraw: $ 300 Withdrawal of $ 300.0 successful. Remaining balance: $ 700.0 Bank ATM Simulator Choices: 1 . Check Balance 2 . Withdraw Funds 3 . Deposit Funds 4 . Exit Enter your choice: 3 Enter the amount to deposit: $ 700 Deposit of $ 700.0 successful. New balance: $ 1400.0 Bank ATM Simulator Choices: 1 . Check Balance 2 . Withdraw Funds 3 . Deposit Funds 4 . Exit |