History of Rock Paper Scissor game
The origins of Rock-Paper-Scissors can be traced back to ancient hand games found in various cultures throughout history. Its exact origins are unclear, but similar hand games were played in China, Japan, and other regions for centuries. The game gained popularity in Japan, where it was known as “Janken.”
In the West, its earliest known mention dates back to the 17th century in Italy. Variants of the game were also documented in historical texts in England and France. The game’s simplicity and ease of play contributed to its widespread adoption and enduring popularity.
What are rules of rock paper scissor game?
- Rock-Paper-Scissors is a hand game played between two people.
- Players simultaneously form one of three shapes:
- Rock,
- Paper
- Scissors.
- Rules of Game
- Rock crushes Scissors
- Scissors cuts Paper
- Paper covers Rock.
- Winners determined based on shape interactions:
- Rock beats Scissors
- Scissors beats Paper
- Paper beats Rock.
- Often used for decision-making or as a simple game of chance.
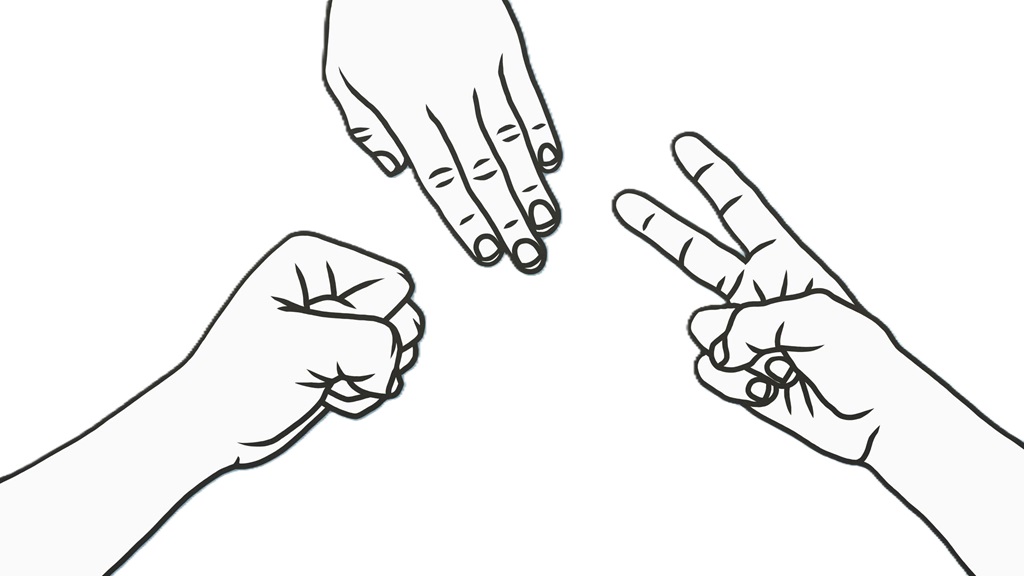
How to create Rock Paper Scissor Game in Java?
- Develop a function for player input
- Rock
- Paper
- Scissors
- Use Java Scanner class to get player input and other player would be computer.
- We would randomly generate value for Computer Player.
- So, one player is giving input from console & other randomly getting generated.
- Randomly select the choice using Random Class in Java
- Create comparison rules to determine which player is winner.
- Use conditional statements for comparison.
- Player has any of following combination would WIN:
- Player1 having Rock & Player2 has Paper -> Player 1 Wins
- Player1 having Paper & Player2 has Scissor -> Player 1 Wins
- Player1 having Scissor & Player2 has Rock. -> Player 1 Wins
- Otherwise, Player2 Wins (if it’s not tie)
- Display outcomes for players:
- Win, Lose, or Tie.
- Present both player and computer choices for clarity.
- Implement a loop for game continuation, if desired by the player.
Play Rock Paper Scissor Game in Java
import java.util.Random; import java.util.Scanner; enum Choice { ROCK, PAPER, SCISSORS; public static Choice getRandom() { return values()[new Random().nextInt(values().length)]; } } public class RockPaperScissors { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); Choice[] choices = Choice.values(); while (true) { System.out.println("Enter your choice (ROCK, PAPER, or SCISSORS): "); String userInput = scanner.nextLine().toUpperCase(); Choice playerChoice; try { playerChoice = Choice.valueOf(userInput); } catch (IllegalArgumentException e) { System.out.println("Invalid input. Please enter ROCK, PAPER, or SCISSORS."); continue; } Choice computerChoice = Choice.getRandom(); System.out.println("Your choice: " + playerChoice); System.out.println("Computer's choice: " + computerChoice); if (playerChoice == computerChoice) { System.out.println("It's a tie!"); } else if ((playerChoice == Choice.ROCK && computerChoice == Choice.SCISSORS) || (playerChoice == Choice.PAPER && computerChoice == Choice.ROCK) || (playerChoice == Choice.SCISSORS && computerChoice == Choice.PAPER)) { System.out.println("You win!"); } else { System.out.println("Computer wins!"); } System.out.println("Do you want to play again? (yes/no)"); String playAgain = scanner.nextLine().toLowerCase(); if (!playAgain.equals("yes")) { break; } } scanner.close(); } }
Output: Created Rock Paper Scissor Game in Java
Enter your choice (ROCK, PAPER, or SCISSORS): rock Your choice: ROCK Computer's choice: SCISSORS You win! Do you want to play again? (yes/no) yes Enter your choice (ROCK, PAPER, or SCISSORS): paper Your choice: PAPER Computer's choice: PAPER It's a tie! Do you want to play again? (yes/no) no