History Of Hangman Game?
The history of Hangman dates back to the 18th century, believed to have originated in Europe. It’s a traditional paper-and-pencil guessing game where one player thinks of a word and draws a blank line for each letter of that word. The other player guesses letters to fill in the blanks, attempting to solve the word within a limited number of incorrect guesses. If the guesser fails to solve the word before completing a drawing of a hanging man, they lose.
Though its origins are unclear, Hangman has been widely popularized as a word game in different cultures and has appeared in various formats and adaptations across the world. It’s commonly used for educational purposes, entertainment, and language learning, continuing to be a fun and challenging pastime for players of all ages.
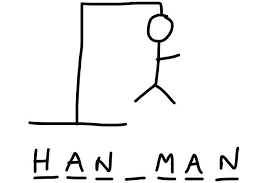
How to create Basic Hangman Game in Java?
- Accept secret word input from the console using Scanner class.
- We will enter secret word from console
- Initialize the game with maximum attempts.
- Also, Initialize an empty array for guessed letters.
- Display underscores for each letter in the secret word.
- Allow the player to input a letter.
- Handle guesses.
- Check if the guessed letter matches any in the secret word
- If matched, then update the displayed word accordingly.
- Otherwise, Reduce attempts for incorrect guesses.
- Repeat until the word is guessed or attempts run out.
- Display success or failure messages based on the game’s outcome.
Program: Create Basic Hangman Game in Java
import java.util.Scanner; public class HangmanGame { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); System.out.print( "Enter the secret word: " ); String secretWord = scanner.nextLine().toLowerCase(); // Take the secret word as input int attempts = 6 ; // Set maximum attempts StringBuilder guessedLetters = new StringBuilder(); char [] wordToGuess = new char [secretWord.length()]; for ( int i = 0 ; i < secretWord.length(); i++) { wordToGuess[i] = '_' ; // Initialize wordToGuess with underscores } boolean gameWon = false; while (attempts > 0 && !gameWon) { System.out.println( "Word to guess: " + String.valueOf(wordToGuess)); System.out.println( "Attempts left: " + attempts); System.out.print( "Enter a letter: " ); char guess = scanner.next().toLowerCase().charAt( 0 ); // Take a letter as input if (guessedLetters.toString().indexOf(guess) != - 1 ) { System.out.println( "You already guessed that letter!" ); continue ; } guessedLetters.append(guess); boolean letterFound = false ; for ( int i = 0 ; i < secretWord.length(); i++) { if (secretWord.charAt(i) == guess) { wordToGuess[i] = guess; letterFound = true ; } } if (!letterFound) { attempts--; } if (String.valueOf(wordToGuess).equals(secretWord)) { gameWon = true ; } } if (gameWon) { System.out.println( "Congratulations! You guessed the word: " + secretWord); } else { System.out.println( "Sorry, you've run out of attempts. The word was: " + secretWord); } scanner.close(); } } |
Output: Play Basic Hangman game in Java
Word to guess: ________ Attempts left: 6 Enter a letter: l Word to guess: l_______ Attempts left: 6 Enter a letter: e Word to guess: l__r___g Attempts left: 6 Enter a letter: a Word to guess: l__r__ng Attempts left: 6 Enter a letter: r Word to guess: l__rning Attempts left: 6 Enter a letter: n Congratulations! You guessed the word: Learning |