- Given a base & concrete class in java.
- Base class is not Serializable
- Concrete class implements Serializable interface and extending its base class
- Base class must have default or no arg constructor.
- We have discussed
- Impact of Inheritance on serialization in java, when base class is serializable.
Impact of serialization on inheritance in java
1.) What happens, when concrete class is serialized?
- Date members of concrete class will get serialized
- Date members of base class will not get serialized
2.) What happens, when concrete class is deserialized?
- Data members of concrete class gets deserialized
- Data members of base class get default value
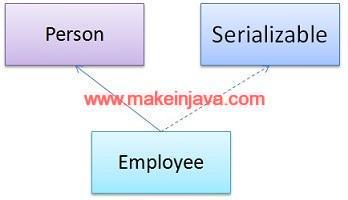
Code: Serialization when concrete class is serializable
1.) Person (Base) class (is not serializable)
- Person class does not implements Serializable interface.
- Data members of Person class will not be serialized.
package org.learn; public class Person { public String firstName; public int age; public String contact; public Person(String firstName, int age, String contact) { this .firstName = firstName; this .age = age; this .contact = contact; } public Person() { } public String toString() { return "[" + firstName + " " + age + " " +contact + "]" ; } } |
2.) Employee (Concrete) class implements Serializable interface
- Employee class implements Serializable marker interface
- Employee class extends Person class.
- When we will serialize the Employee object
- Data member pertaining to employee object will gets serialized
- Data members of person class will not get serialized.
- When we deserialize the employee object
- Employee data members will gets deserialized.
- Person data members will get default values (will not get serialized).
package org.learn; import java.io.Serializable; public class Employee extends Person implements Serializable { private static final long serialVersionUID = 1L; public String department; public int employCode = 1010 ; public int salary; public Employee(String department, int salary, int employCode, String firstName, int age, String contact) { super (firstName,age, contact); this .department = department; this .salary = salary; this .employCode = employCode; } public String toString() { return super .toString() + "[" + department + " " + employCode + " " + salary + "]" ; } } |
3.) SerializeDeserialize class
- SerializeDeserialize class contains main function.
- SerializeDeserialize class will serialize and deserialize the Employee object.
package org.learn; import java.io.File; import java.io.FileInputStream; import java.io.FileOutputStream; import java.io.IOException; import java.io.ObjectInputStream; import java.io.ObjectOutputStream; public class SerializeDeserialize { public static void serialize() throws IOException { Employee employee = new Employee( "IT" , 25 , 9999 , "Mike" , 34 , "001894536" ); //Set static variable FileOutputStream output = new FileOutputStream( new File( "savePerson.txt" )); ObjectOutputStream outputStream = new ObjectOutputStream(output); outputStream.writeObject(employee); output.close(); outputStream.close(); System.out.println( "Serialized the employee object : " +employee); } public static void deSerialize() throws IOException, ClassNotFoundException { FileInputStream inputStream = new FileInputStream( new File( "savePerson.txt" )); ObjectInputStream objectInputStream = new ObjectInputStream(inputStream); Employee employee = (Employee) objectInputStream.readObject(); System.out.println( "Deserialize the employee object :" + employee); objectInputStream.close(); inputStream.close(); } public static void main(String[] args) throws IOException, ClassNotFoundException { serialize(); deSerialize(); } } |
Output: Serialization in java, drive class is serializable
Serialized the employee object : [Mike 34 001894536][IT 9999 25] Deserialize the employee object :[null 0 null][IT 9999 25] |